Using Native Lazy loading with IOLazy
setup
Grab the latest release from io-lazyload releases
Or install it through NPM with npm install iolazyload
add some inline javascript (or in your bundle however you choose) to check for the loading attribute, then apply our scripts.
for every image you wish to lazyload add a data-src
attribute, a data-srcset
for your responsive images and the loading='lazy'
attribute.
Javascript Needed:
<script>
if ('loading' in HTMLImageElement.prototype) {
// you could also use the class/id you use for the IOLazy library here for the images variable.
const images = document.querySelectorAll("img");
images.forEach( img => {
img.src = img.dataset.src;
// this if clause is only needed if you have __ANY__ images with a srcset attribute.
if (img.dataset.srcset) {
img.srcset = img.dataset.srcset;
}
});
} else {
let scriptEl = document.createElement('script');
// set the scripts attribute to 'async'
scriptEl.async = true;
// set the src of the script element to the IOlazy javascript file.
script.src = './path/to/iolazy.min.js';
// append this newly created script element to the end of the body of our page.
document.appendChild(scriptEl);
}
</script>
Markup needed for your images
Add the data-src
, data-srcset
and
loading
attributes
<img sizes="(min-width: 530px) 25vw, 100vw"
data-srcset="your/image-177w.png 177w,
your/image-240w.png 240w,
your/image-321w.png 321w"
src="your/low/quality/placeholder.png"
alt="Your Alt Text"
class="lazyload"
loading="lazy"
>
example
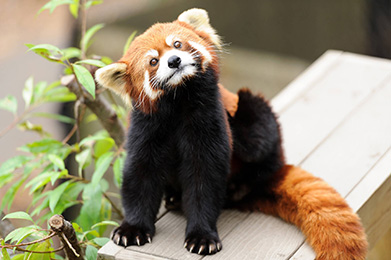
Red Panda Stretching By Eric Kilby
Troubleshooting
Internet Explorer
In order for the above check for loading in your page there is an arrow function used. Specifically:
images.forEach( img => { // this arrow function here
img.src = img.dataset.src;
// this if clause is only needed if you have __ANY__ images with a srcset attribute.
if (img.dataset.srcset) {
img.srcset = img.dataset.srcset;
}
});
You'll need to swap the arrow function out if you wish to support browsers that don't understand arrow functions. This can either be done inline or through your build step.
images.forEach( function (img) {
img.src = img.dataset.src;
if (img.dataset.srcset) {
img.srcset = img.dataset.srcset;
}
});
Images wont show up and I've got all the right code
You should double check to make sure you have a src
attribute on your image tage. Some browsers wont swap out the newly swapped in image without an image or placeholder.